Subscriptions API
Subscriptions allow merchants to create subscription agreements and automatically collect the payment amount from the customer on a recurring basis.
Account configuration is required before live transactions
We need to configure your account before you can start accepting live payments. Kindly message [email protected] to request for key configuration. Your account's eligibility will be reviewed before you can access this feature.
Integrating Subscriptions through the Payment Intent Workflow
The payment intent workflow is the main workflow used to make payments via PayMongo. To enable subscriptions, a few additional steps just need to be added to the existing workflow.
1. Creating a plan
Before being able to create subscriptions, you have to first determine the subscription plans that you’re offering. Plans define the essential elements of the products like amount, currency, and cycle. Your customers’ subscriptions will then be based on the details of the plans you created.
You only need to do this during the initial integration. You do not need to create a new plan for every new subscription. 1 plan can be linked to multiple subscriptions. On a macro level, this is how it looks like:
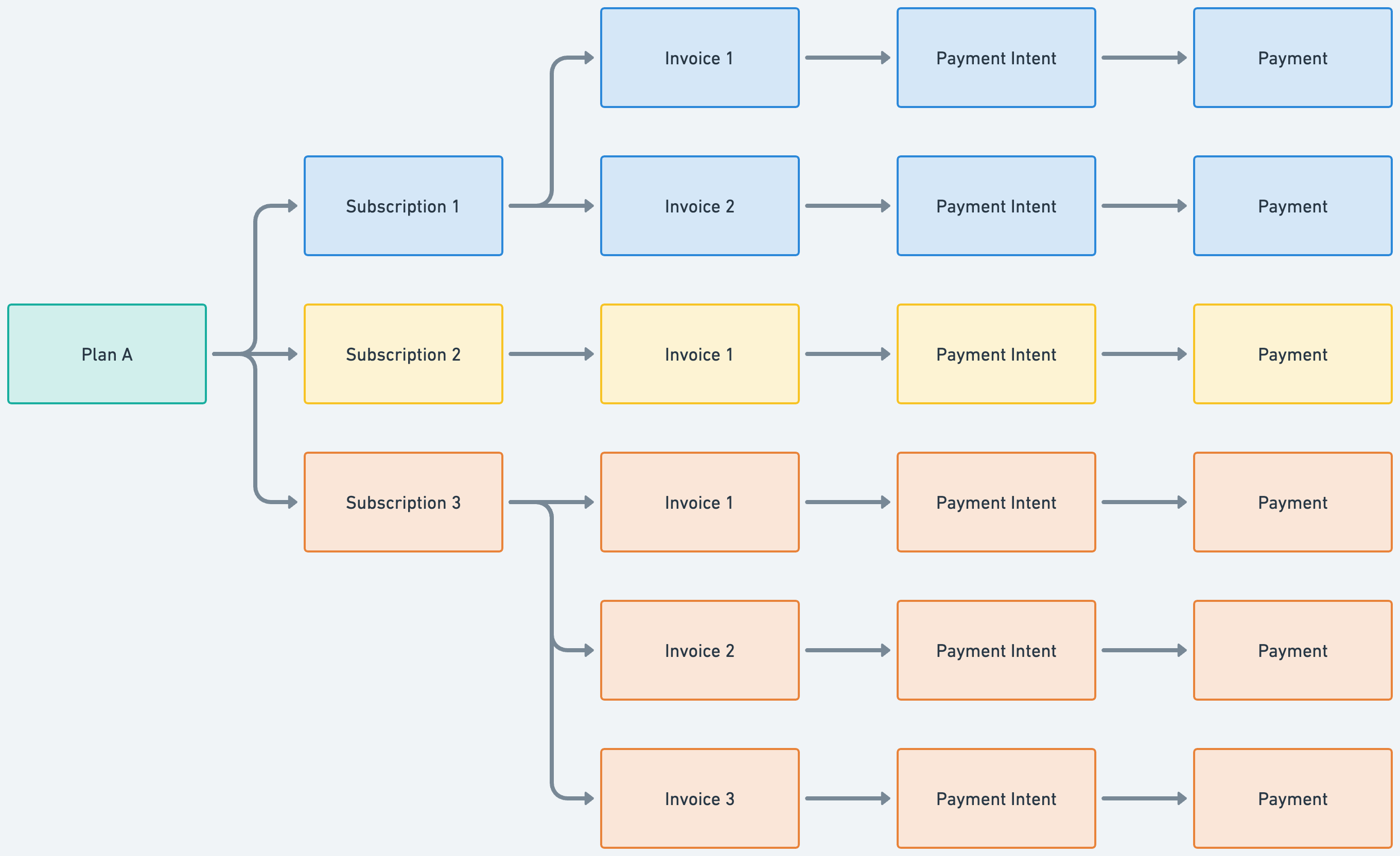
You can use the Create a Plan endpoint and send the following attributes:
{
“name”: “Basic Plan”,
“description”: “The basic plan offers essential coverage for life’s unexpected events.”,
“amount”: “100000”,
“currency”: “PHP”,
“interval”: “monthly”,
“interval_count”: “1”,
“cycle_count”: “6”, //optional (If not provided, plan will run indefinitely.)
}
Make sure to save the plan ID so you can use it when creating subscriptions on the next step!
You can update the details later on using the Update a Plan endpoint if you decide to make minor changes to the products that you’re offering. For now, you have the ability to update the plan name
, description
, and amount
only. Take note that changing the plan details will affect all the connected subscriptions’ next invoice generation so make sure your customers are aware before applying changes to all your existing plans! All existing invoices will not be updated.
2. Creating a subscription
Based on the plans you offer, you can now create a subscription for your customer depending on their chosen plan. A subscription will always be linked to a plan, which contains the payment details.
-
A customer represents your customer in our database. You have to create a customer to be able to link a subscription to your customer. If the customer is an existing one, you can use the same customer ID.
-
Based on your customer’s selected plan, you can create a subscription by passing the following attributes:
{ “customer_id”: “cus_Exy3jegPk4eEagpQcE6wnLB4”, “plan_id”: “plan_ajeDG2y6WgnrCXaamWFmPUw2” }
Make sure to store the Payment Intent ID (
latest_invoice.payment_intent.id
) from the response, you will need this when attaching the payment method on the succeeding steps. The invoice is automatically generated by us upon successful creation of the subscription. -
The creation follows the same process as normal in the PIPM workflow. For now, we only support card payment method for subscriptions. You have to create a new payment method for every new subscription.
-
The attachment follows the same process as normal in the PIPM workflow. In this step, you will pay for the latest invoice using the payment intent ID generated from Step 2.
Take note that your customer needs to make the payment within 24 hours. Otherwise, we will automatically cancel the created subscription.
-
Redirecting the customer for authentication
The authentication follows the same process as normal in the PIPM workflow.
That’s it! When the first payment of the subscription is successful, a payment is created and the subscription is activated. We will then automatically process the succeeding payments of the subscription on the next billing schedule.
If the final amount is variable, you can add or deduct an amount to adjust the final amount to be billed on the next invoice using the Create an Invoice Line Item endpoint. You can do this before the next generated invoice is finalized.
Canceling a subscription*
You have the ability to manually cancel a subscription at any time. You would usually use this when your customer decides to unsubscribe from the service, subscription period has lapsed, or your customer still fails to pay the latest invoice of the subscription due to problems with the payment method (e.g. expired card, insufficient funds, etc). You can use the Cancel a Subscription endpoint to do this.
Take note that once the subscription has been canceled, it will take effect immediately and no further invoices will be generated. However, if there is an existing invoice that is not paid (invoice
status
is open), you can process the manual capture of the payment using the Pay an Open Invoice endpoint.
We currently do not support prorating. The full amount of the subscription will remain charged to the customer.
Changing the subscription’s plan*
If your customer wishes to upgrade or downgrade their subscription plan, you can use the Change a Subscription's Plan endpoint to do this.
The new plan will take effect on the next billing schedule of the subscription. This means, the current billing cycle will remain in effect until the next billing schedule. On the next billing schedule, a new invoice will be automatically generated following the new plan details.
Take note that once an invoice has been generated, any changes on the subscription’s plan will take effect on the next invoice that will be generated.
Changing the subscription’s payment method*
Your customer can change the linked payment method in case it does not have sufficient funds or has expired. If this happens, your customer needs to be authenticated again.
-
The creation follows the same process as normal in the PIPM workflow. For now, we only support card payment method for subscriptions.
-
Update the Payment Method of the Subscription
You need to update the subscription’s payment method to the newly created payment method. If the status of the
setup_intent
from the response is awaiting_next_action, you can proceed to the next step. Otherwise, you have to repeat Step 1.Make sure to store the
setup_intent.next_action_url
, you will need this on the next step. -
Redirecting the customer for authentication
You must render the
setup_intent.next_action_url
to authenticate the cardholder.
When changing the card details, we will just authorize an amount from the cardholder and then immediately cancel the amount after successful authentication. The purpose of this is to authenticate the card of your customer so you can collect the payment for the next or any open invoices. For any open invoices where the subscription is past_due, unpaid or cancelled, you can collect your customer’s payment using the Pay an Open Invoice endpoint.
*Cancelling or changing the subscription plans and payment methods are steps that are optional but highly recommended.
Triggering a Test Subscription Cycle*
For test subscriptions (in test mode), you now have the ability to trigger a new subscription cycle via the trigger a new test subscription cycle endpoint. You will usually use this to trigger a new subscription cycle for an existing subscription, removing the wait time dictated by the interval attribute.
Note: This feature can only be used for TEST MODE subscriptions
Lifecycle
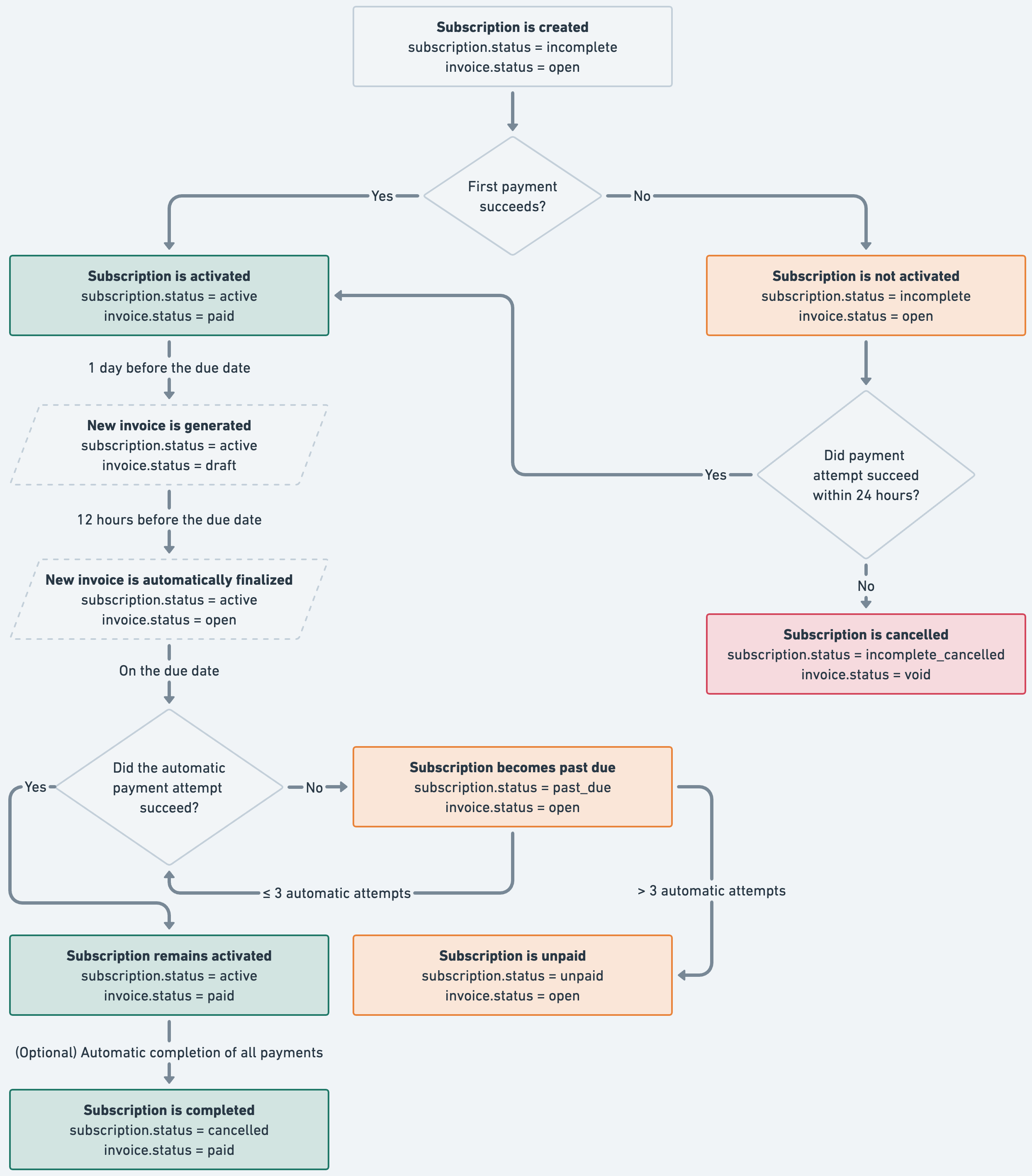
Invoice
Invoices serve as a reference for the subscription payment details of a specific cycle, which are automatically generated from an active subscription.
Lifecycle
- On the first payment, an invoice is automatically generated after the subscription has been successfully created. Your customer needs to settle the invoice payment within 24 hours. Otherwise, both the subscription and invoice will be canceled.
- On the succeeding payments, an invoice is automatically generated 1 day before an active subscription's next billing schedule, where the
status
is set to draft. At this point, you can still add or deduct anamount
to adjust the final invoice amount to be billed using the Create an Invoice Line Item endpoint. - PayMongo automatically updates the invoice
status
to open after 12 hours. This is to finalize the invoice information so PayMongo can process the payment accordingly. - PayMongo will automatically attempt to pay the invoice using the customer's saved card on its due date.
- If the payment succeeds, the invoice
status
is updated to paid. - If the payment fails, the invoice
status
remains open.
- If the payment succeeds, the invoice
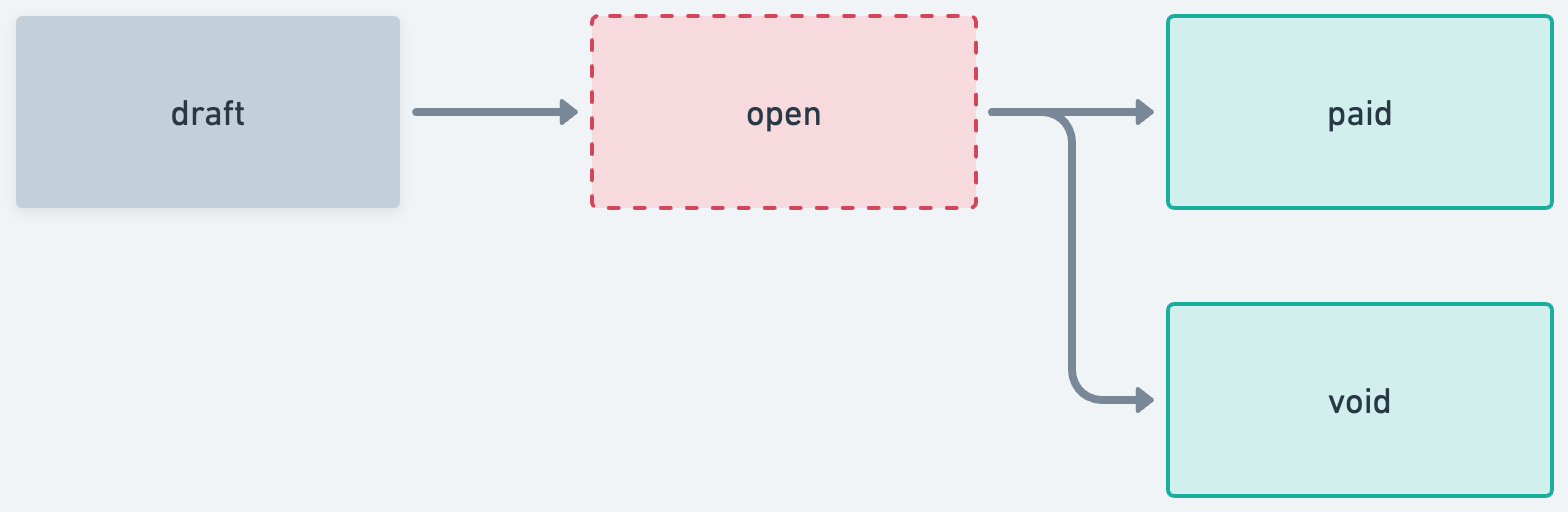
Webhook Events
Get notified when you have successful or failed subscriptions by using webhooks. To monitor the transition of the Subscriptions and Invoices from your backend, you could be notified by listening to the events by registering your webhook endpoint using our Webhook API.
Webhooks
You should not create a webhook in your code. You can try using API tools such as Postman or curl to create your webhook once. Please take note that you should not create multiple webhooks for every source that will be created. One webhook event is enough to receive the necessary information.
Events for subscription and invoices:
Events | Description |
---|---|
subscription.past_due | Event is sent when the subscription’s status is updated to past_due . |
subscription.unpaid | Event is sent when the subscription’s status is updated to unpaid . |
subscription.activated | Event is sent when the subscription’s status is updated to active . |
subscription.updated | Event is sent when the subscription’s status is updated to incomplete_cancelled or cancelled . |
subscription.invoice.created | Event is sent when a new invoice was successfully created. |
subscription.invoice.finalized | Event is sent when the new invoice has been finalized and is awaiting payment. |
subscription.invoice.paid | Event is sent when the payment attempt for the invoice succeeded. |
subscription.invoice.payment_failed | Event is sent when the payment attempt for the invoice failed. |
Event notifications will be sent to your registered webhook endpoint containing relevant information as shown below:
{
"data": {
"id": "evt_RTvNR19hqPQAroiVRmhsuFZV",
"type": "event",
"attributes": {
"type": "subscription.updated",
"livemode": true,
"data": {
"id": "subs_udczMB4j6VQ372yxcffhfxf8",
"type": "subscription",
"attributes": {
"id": "subs_udczMB4j6VQ372yxcffhfxf8",
"customer_id": "cus_Y4i5V1KSbrseGG9Vy91G4KCZ",
"default_customer_payment_method_id": null,
"livemode": true,
"organization_id": "org_R6sN7BSxAbUsYCReUSqPKELD",
"plan_id": "plan_6GwwfH3jp1KBuYvMykVhsQ13",
"status": "incomplete_cancelled",
"cancelled_at": 1701665145,
"created_at": 1701665132,
"next_billing_schedule": null,
"updated_at": 1701665145
}
},
"previous_data": {},
"created_at": 1701665145,
"updated_at": 1701665145
}
}
}
Updated 29 days ago